Fetching github stars and forks using Github API
- Published on
Introduction
Let's say we want to display the number of stars a repository has on our website. You can see it on my homepage the list of my repositories and the number of stars and forks they have.
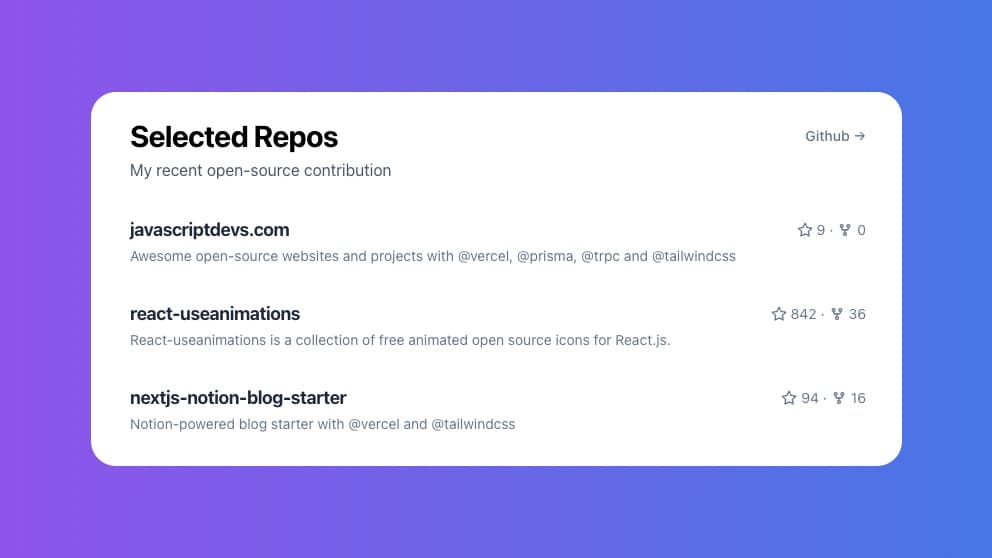
In this tutorial, we will learn how to retrieve the number of stars a repository has using the GitHub API and JavaScript.
Making a request to the GitHub API
Now that we have our Personal Access Token, we can start making requests to the GitHub API.
To retrieve the number of stars a repository has, we can use the https://api.github.com/repos/{owner}/{repo}
endpoint. This endpoint requires the owner and repository name as path parameters, and returns a JSON object with information about the repository, including the number of stars.
Here is an example of how to make a request to this endpoint using the fetch() function in JavaScript:
const token = 'YOUR_TOKEN_HERE'
async function getRepoStarsAndForks(owner, repo) {
const endpoint = `https://api.github.com/repos/${owner}/${repo}`
const headers = {
Authorization: `Token ${token}`,
}
try {
const response = await fetch(endpoint, { headers })
const data = await response.json()
return {
stars: data.stargazers_count,
forks: data.forks_count,
}
} catch (error) {
console.error(error)
}
}
// Usage
const repoDetail = await getRepoDetail('tuanphungcz', 'javascriptdevs.com')
console.log('Stars: ', repoDetail.stars) // Stars: 94
console.log('Forks: ', repoDetail.forks) // Forks: 24
This function takes the owner and repo parameters, which should be the owner's username and the repository name respectively. It then makes a GET request to the /repos/{owner}/{repo}
endpoint with the Authorization header set to our Personal Access Token.
If the request is successful, the function returns the number of stars the repository has. If there is an error, it logs the error to the console.
Use it in your Next.js app
Here is an example of how to use this function in my blog app, where I'm fetching the number of stars and forks for my github repositories.
export async function getStaticProps(context) {
const repos = [
{
owner: 'tuanphungcz',
repo: 'javascriptdevs.com',
},
{
owner: 'tuanphungcz',
repo: 'phung.io',
},
]
const reposWithGithubData = await Promise.all(
repos.map(async (repo) => {
const repoDetail = await getRepoStarsAndForks(repo.owner, repo.repo)
return { ...repo, stars: repoDetail.stars, forks: repoDetail.forks }
})
)
return {
props: {
reposWithGithubData,
},
}
}
Rate limits
There are rate limits on the GitHub API. The rate limit is 60 requests per hour for unauthenticated requests, and 5000 requests per hour for authenticated requests.
Setting up a GitHub Personal Access Token
In order to make requests to the GitHub API, we will need to authenticate ourselves. One way to do this is by using a Personal Access Token.
To create a Personal Access Token, follow these steps:
- Go to your GitHub Settings.
- Click on the
Developer Settings
tab. - Click on the
Personal Access Tokens
tab. - Click on the
Generate new token
button. - Enter a name for the token and select the
repo
scope. - Click on the
Generate token
button. - Keep this token safe, as it can be used to access your repositories.
Thanks for reading
Let me know in the comments section what you think about this article. If you love it, you know what to do! Share it with your friends and colleagues.
If you want me to cover some topics in the next post, DM me on twitter @tuanphung_, or if you have any suggestions, feel free to comment below.
See ya next time and keep on hacking ✌